Note
Click here to download the full example code
Retime an one dimensional path¶
Import necessary libraries.
7 import toppra as ta
8 import toppra.constraint as constraint
9 import toppra.algorithm as algo
10 import numpy as np
11 import matplotlib.pyplot as plt
12
13 ta.setup_logging("INFO")
We now generate a simply path. When constructing a path, you must “align” the waypoint properly yourself. For instance, if the waypoints are [0, 1, 10] like in the above example, the path position should be aligned like [0, 0.1, 1.0]. If this is not done, the CubicSpline Interpolator might result undesirable oscillating paths!
Setup the velocity and acceleration
Setup the problem instance and solve it.
38 instance = algo.TOPPRA([pc_vel, pc_acc], path, solver_wrapper='seidel')
39 jnt_traj = instance.compute_trajectory(0, 0)
Out:
INFO [algorithm.py : 104] No gridpoint specified. Automatically choose a gridpoint with 129 points
INFO [algorithm.py : 191] Successfully parametrize path. Duration: 4.084, previously 1.000)
INFO [algorithm.py : 193] Finish parametrization in 0.006 secs
We can now visualize the result
43 duration = jnt_traj.duration
44 print("Found optimal trajectory with duration {:f} sec".format(duration))
45 ts = np.linspace(0, duration, 100)
46 fig, axs = plt.subplots(3, 1, sharex=True)
47 qs = jnt_traj.eval(ts)
48 qds = jnt_traj.evald(ts)
49 qdds = jnt_traj.evaldd(ts)
50 axs[0].plot(ts, qs)
51 axs[1].plot(ts, qds)
52 axs[2].plot(ts, qdds)
53 plt.show()
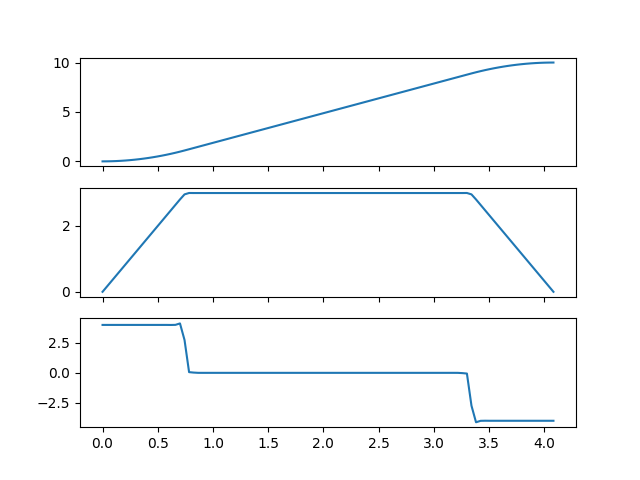
Out:
Found optimal trajectory with duration 4.083554 sec
Total running time of the script: ( 0 minutes 0.634 seconds)