Note
Click here to download the full example code
Retime a path subject to robust kinematic constraintsΒΆ
5 import toppra as ta
6 import toppra.constraint as constraint
7 import toppra.algorithm as algo
8 import numpy as np
9 import matplotlib.pyplot as plt
10 import argparse
11
12
13 parser = argparse.ArgumentParser(
14 description="An example showcasing the usage of robust constraints."
15 "A velocity constraint and a robust acceleration constraint"
16 "are considered in this script."
17 )
18 parser.add_argument(
19 "-N",
20 "--N",
21 type=int,
22 help="Number of segments in the discretization.",
23 default=100,
24 )
25 parser.add_argument("-v", "--verbose", action="store_true", default=False)
26 parser.add_argument("-du", "--du", default=1e-3, type=float)
27 parser.add_argument("-dx", "--dx", default=5e-2, type=float)
28 parser.add_argument("-dc", "--dc", default=9e-3, type=float)
29 parser.add_argument("-so", "--solver_wrapper", default="ecos")
30 parser.add_argument("-i", "--interpolation_scheme", default=1, type=int)
31 args = parser.parse_args()
32 if args.verbose:
33 ta.setup_logging("DEBUG")
34 else:
35 ta.setup_logging("INFO")
36
37 # Parameters
38 N_samples = 5
39 dof = 7
40
41 # Random waypoints used to obtain a random geometric path.
42 np.random.seed(9)
43 way_pts = np.random.randn(N_samples, dof)
44
45 # Create velocity bounds, then velocity constraint object
46 vlim_ = np.random.rand(dof) * 20
47 vlim = np.vstack((-vlim_, vlim_)).T
48 # Create acceleration bounds, then acceleration constraint object
49 alim_ = np.random.rand(dof) * 2
50 alim = np.vstack((-alim_, alim_)).T
51
52 path = ta.SplineInterpolator(np.linspace(0, 1, 5), way_pts)
53 pc_vel = constraint.JointVelocityConstraint(vlim)
54 pc_acc = constraint.JointAccelerationConstraint(
55 alim, discretization_scheme=constraint.DiscretizationType.Interpolation
56 )
57 robust_pc_acc = constraint.RobustLinearConstraint(
58 pc_acc, [args.du, args.dx, args.dc], args.interpolation_scheme
59 )
60 instance = algo.TOPPRA(
61 [pc_vel, robust_pc_acc],
62 path,
63 gridpoints=np.linspace(0, 1, args.N + 1),
64 solver_wrapper=args.solver_wrapper,
65 )
66
67 X = instance.compute_feasible_sets()
68 K = instance.compute_controllable_sets(0, 0)
69
70 _, sd_vec, _ = instance.compute_parameterization(0, 0)
71
72 X = np.sqrt(X)
73 K = np.sqrt(K)
75 plt.plot(X[:, 0], c="green", label="Feasible sets")
76 plt.plot(X[:, 1], c="green")
77 plt.plot(K[:, 0], "--", c="red", label="Controllable sets")
78 plt.plot(K[:, 1], "--", c="red")
79 plt.plot(sd_vec, label="Velocity profile")
80 plt.legend()
81 plt.title("Path-position path-velocity plot")
82 plt.show()
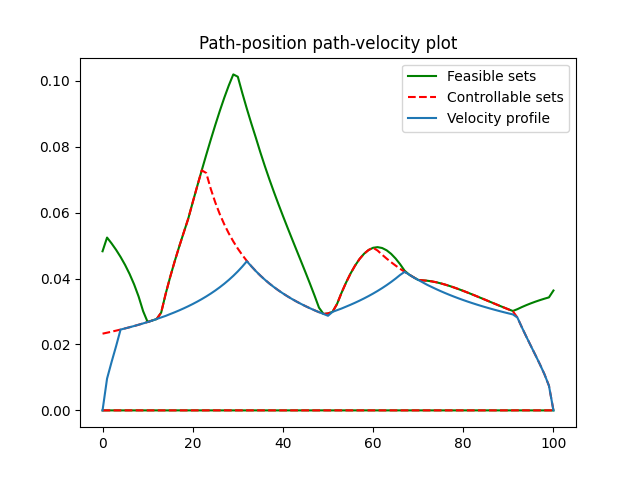
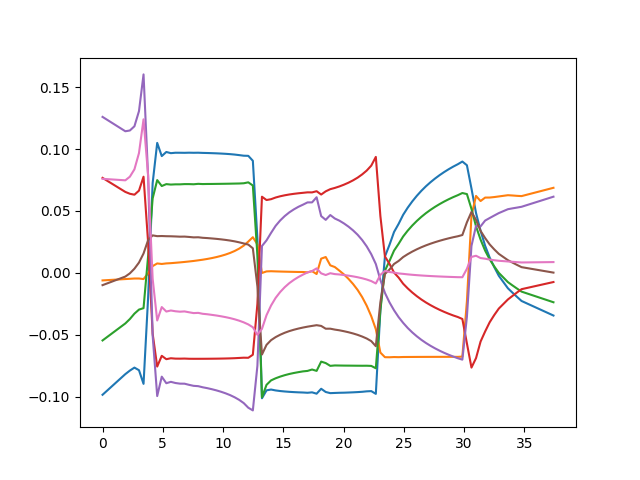
Out:
INFO [algorithm.py : 191] Successfully parametrize path. Duration: 37.417, previously 1.000)
INFO [algorithm.py : 193] Finish parametrization in 1.927 secs
Total running time of the script: ( 0 minutes 6.820 seconds)