Note
Click here to download the full example code
Retime a path to a specific durationΒΆ
toppra allows user to parametrize a path to a specific duration while still satisfy constraints. We will see how to do this in this example. First, import necessary libraries.
9 import toppra as ta
10 import toppra.constraint as constraint
11 import toppra.algorithm as algo
12 import numpy as np
13 import matplotlib.pyplot as plt
14 import time
15
16 ta.setup_logging("INFO")
Parameters
Random waypoints used to obtain a random geometric path. Here, we use spline interpolation.
27 np.random.seed(SEED)
28 way_pts = np.random.randn(N_samples, dof)
29 path = ta.SplineInterpolator(np.linspace(0, 1, 5), way_pts)
Create velocity bounds, then velocity constraint object
Create acceleration bounds, then acceleration constraint object
Setup a parametrization instance
46 instance = algo.TOPPRAsd([pc_vel, pc_acc], path)
47 instance.set_desired_duration(60)
Out:
INFO [algorithm.py : 104] No gridpoint specified. Automatically choose a gridpoint with 290 points
INFO [reachability_algorithm.py : 65] Solver wrapper not supplied. Choose solver wrapper automatically!
INFO [reachability_algorithm.py : 75] Select solver seidel
Retime the trajectory, only this step is necessary.
51 jnt_traj = instance.compute_trajectory(0, 0)
52 ts_sample = np.linspace(0, jnt_traj.get_duration(), 100)
53 qs_sample = jnt_traj(ts_sample, 2)
Out:
INFO [desired_duration_algorithm.py : 158] Desired duration 60.000000 sec is achievable. Continue computing.
INFO [algorithm.py : 191] Successfully parametrize path. Duration: 60.000, previously 1.000)
INFO [algorithm.py : 193] Finish parametrization in 0.048 secs
/home/circleci/repo/toppra/utils.py:23: DeprecationWarning: Call to deprecated function get_duration in module toppra.interpolator.
warnings.warn(
Output
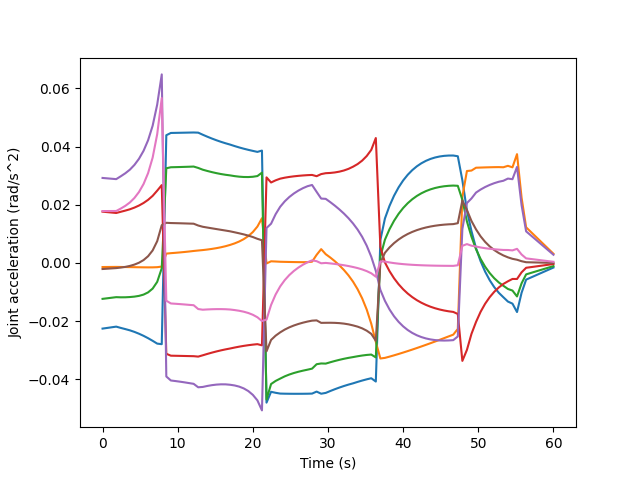
Compute the feasible sets and the controllable sets for viewing. Note that these steps are not necessary.
65 X = instance.compute_feasible_sets()
66 K = instance.compute_controllable_sets(0, 0)
67 _, sd_vec, _ = instance.compute_parameterization(0, 0)
68 X = np.sqrt(X)
69 K = np.sqrt(K)
70 plt.plot(X[:, 0], c='green', label="Feasible sets")
71 plt.plot(X[:, 1], c='green')
72 plt.plot(K[:, 0], '--', c='red', label="Controllable sets")
73 plt.plot(K[:, 1], '--', c='red')
74 plt.plot(sd_vec, label="Velocity profile")
75 plt.title("Path-position path-velocity plot")
76 plt.xlabel("Path position")
77 plt.ylabel("Path velocity square")
78 plt.legend()
79 plt.tight_layout()
80 plt.show()
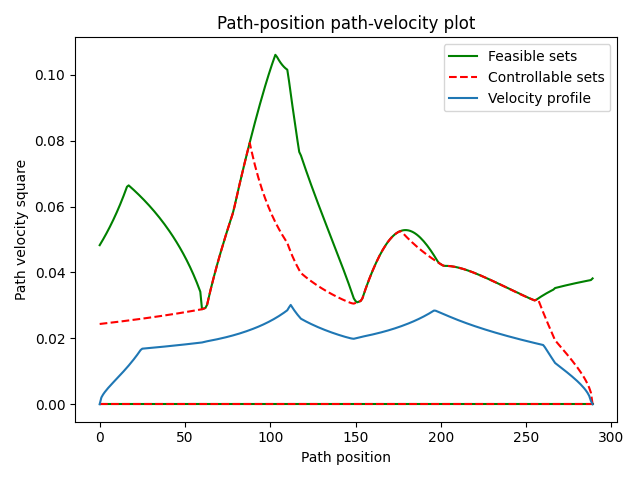
Out:
INFO [desired_duration_algorithm.py : 158] Desired duration 60.000000 sec is achievable. Continue computing.
Total running time of the script: ( 0 minutes 0.458 seconds)